In my recent post Testing: trying to get it right I mentioned that a lot of our tests are of the dirty hybrid kind, somewhere between real Unit tests and real Integration tests. Focusing on the Unit test part, we’re looking into using a mocking framework right now to change the way we write tests – most of all to decouple the different components that we use in the application under test.
Wanting to use the fresh and hype NuGet package manager to install the mocking frameworks, I chose among the ones that were both available there and also looked promising:
Really, it could not have been easier to get all these libraries into the project than using NuGet!
Sample code
So I went ahead and wrote a short and simple test just to get a feel of the syntax they offer. At first I wanted to mock a simple Repository using its interface. Here is what I ended up with:
Telerik JustMock: using syntax from their quick start manual 1: public void MockRepositoryInterfaceTest()
2: {
3: // Arrange
4: var repo = Mock.Create<ITodoRepository>();
5: Mock.Arrange(() => repo.Todos)
6: .Returns(new List<Todo> {new Todo {Description = "my todo"}}.AsQueryable);
7:
8: // Act + Assert
9: repo.Todos.ToList().Count.Should().Be.EqualTo(1);
10: }
Moq: just visit the project homepage
1: public void MockRepositoryInterfaceTest()
2: {
3: // Arrange
4: var repo = new Mock<ITodoRepository>();
5: repo.SetupGet(rep => rep.Todos)
6: .Returns(() => new List<Todo> {new Todo {Description = "my todo"}}.AsQueryable());
7:
8: // Act + Assert
9: repo.Object.Todos.ToList().Count.Should().Be.EqualTo(1);
10: }
FakeItEasy: just visit the project homepage
1: public void MockRepositoryInterfaceTest()
2: {
3: // Arrange
4: var repo = A.Fake<ITodoRepository>();
5: A.CallTo(() => repo.Todos)
6: .Returns(new List<Todo> {new Todo {Description = "my todo"}}.AsQueryable());
7:
8: // Act + Assert
9: repo.Todos.ToList().Count.Should().Be.EqualTo(1);
10: }
In the first test-ride FakeItEasy and JustMock look pretty much identical, whereas the syntax Moq offers is a bit awkward with the SetupGet() method name and the need to call repo.Object to get the instance. I hope to examinate further differences in use as the project moves on.
Mocking concrete classes
Since we’re working with a large application that still has a lot of services and classes not implementing any interface I also wanted to make sure we’ll be able to mock concrete types. Well, this didn’t go so well:
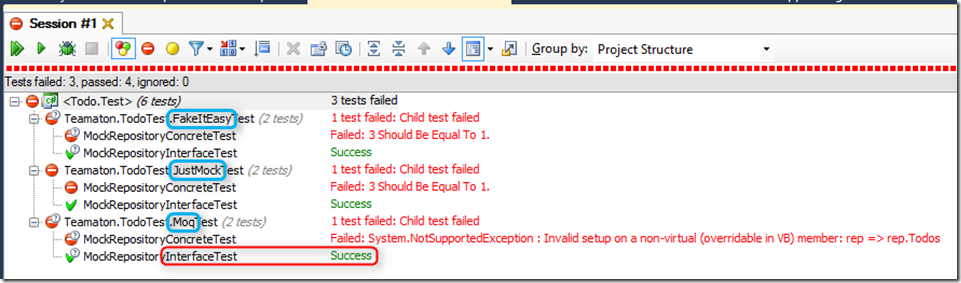
JustMock and FakeItEasy simply returned an instance of the concrete class I gave them, Moq complains that it can’t override the Todos member. So I added the virtual modifier to it and the test is now green. Still, I got the impression that I was trying to do something that I shouldn’t. The following blog post motivates further not to mock concrete classes: Test Smell: Mocking concrete classes. So I guess introducing interfaces as a kind of contract between classes is the way to go, but in the meantime and where we can’t avoid mocking concrete types we’ll be left using Moq.
That’s it for now, happy coding!
Oliver